To start this lab, download this zip file.
Lab 5 - if
, elif
, and else
What Does It Draw?
left-right
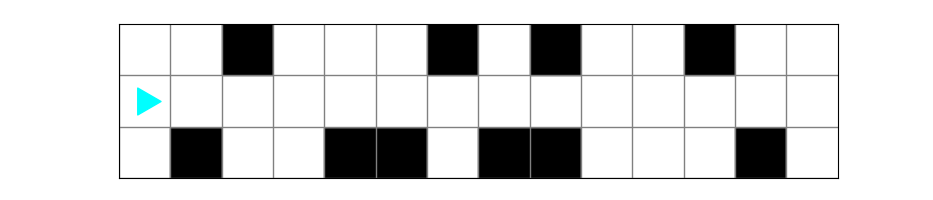
from byubit import Bit
@Bit.run('left-right')
def go(bit):
while bit.front_clear():
bit.move()
if not bit.left_clear():
bit.paint("blue")
elif not bit.right_clear():
bit.paint("green")
else:
bit.paint("red")
Result
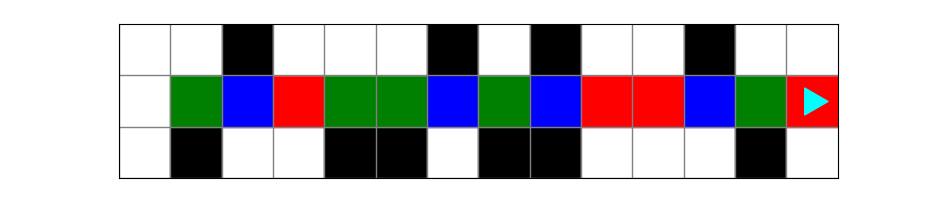
go-left
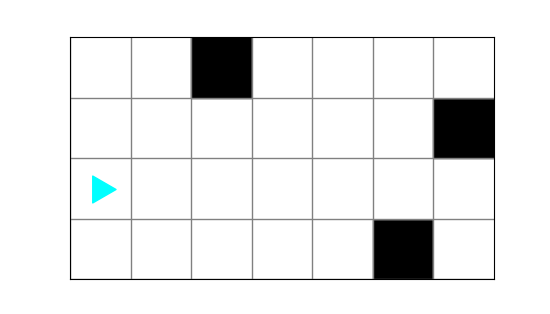
from byubit import Bit
@Bit.run('go-left')
def go(bit):
while bit.front_clear():
bit.move()
if not bit.right_clear():
bit.left()
if bit.is_green():
bit.paint('blue')
else:
bit.paint('green')
Result
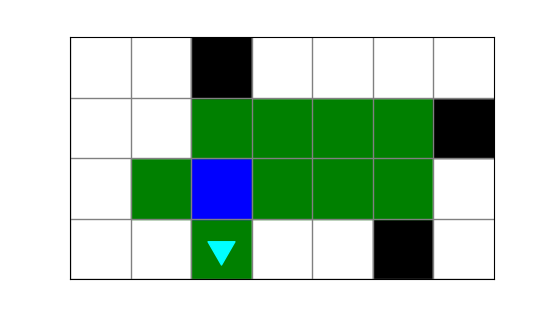
add-color
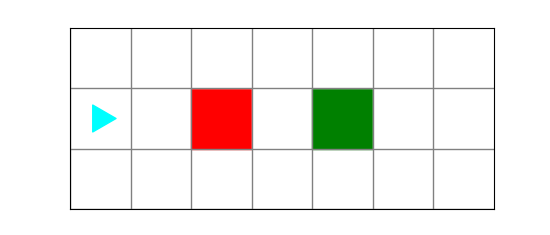
from byubit import Bit
@Bit.run('add-color')
def go(bit):
bit.paint('blue')
while bit.front_clear():
bit.move()
if bit.is_empty():
bit.paint('blue')
Result
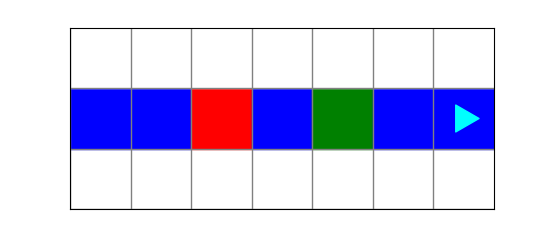
Activities
invert.py
Invert blue squares to empty and empty squares to blue.
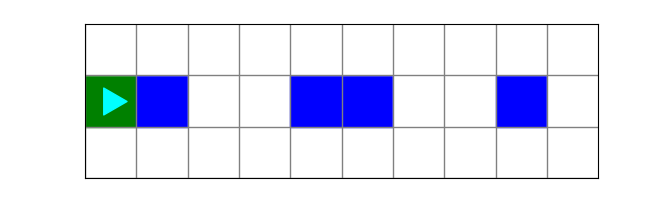
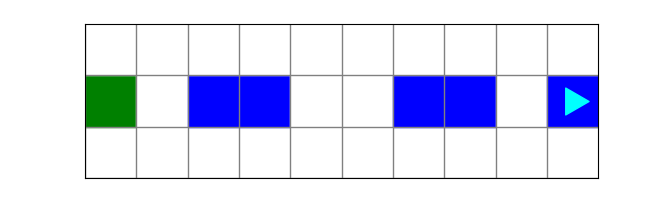
Bit Fact: You can erase the color in a square using bit.erase()
invert_carefully.py
Rules
- Blue => empty
- Empty => blue
- Anything else => leave it alone
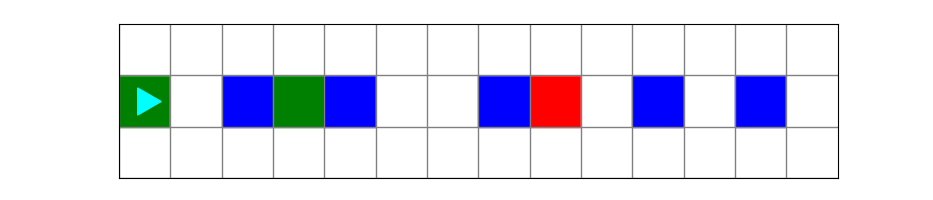
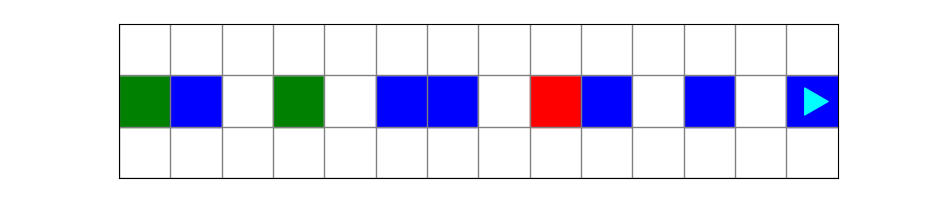
Hint: You can have if
and elif
with no else
block.
wander.py
Bit is restless and wants to go for a walk.
Rules
- Bit moves until it’s blocked in front
- Bit turns left when encountering a green square
- Bit turns right when encountering a blue square
- Bit paints empty squares red
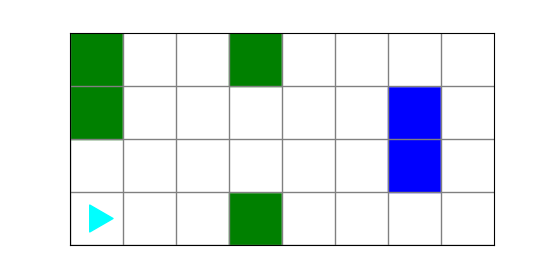
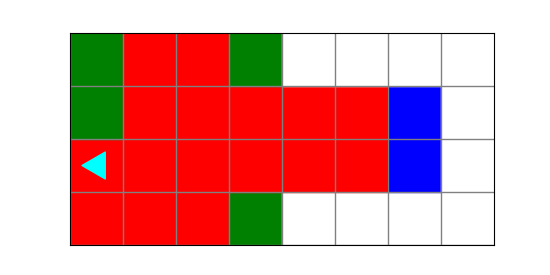
fix_reds.py
Turn any red square AFTER the green square into a blue square.
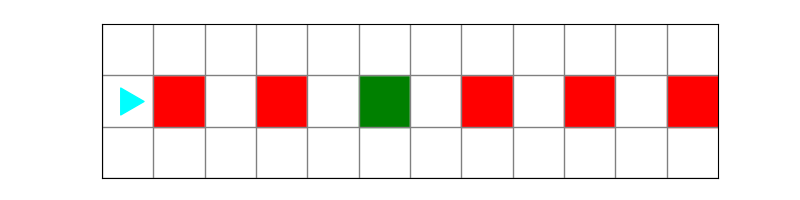
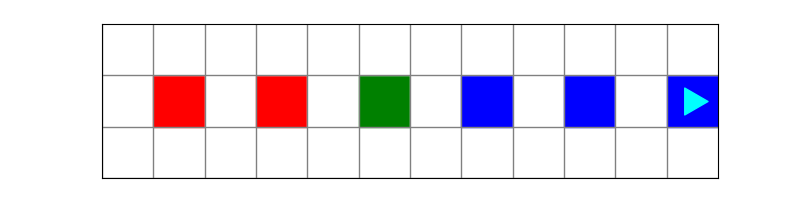
waterfall.py
Bit is a rushing river!
The water always moves forward, but if there is no ground beneath, it drops!
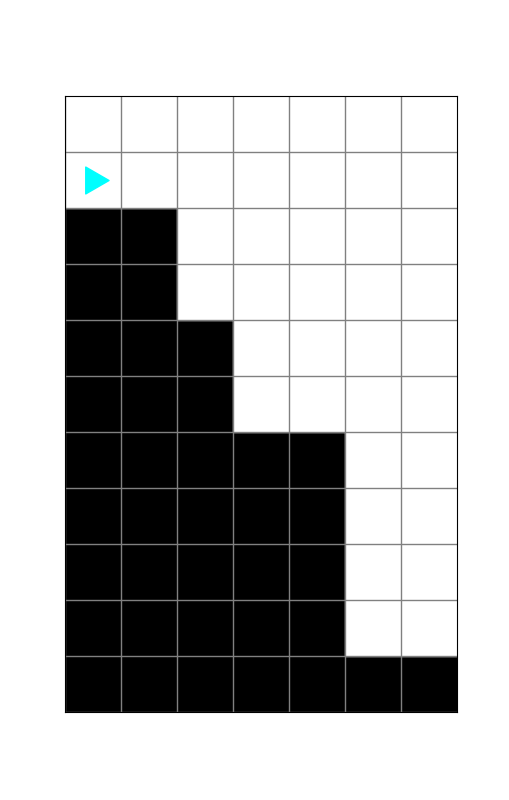
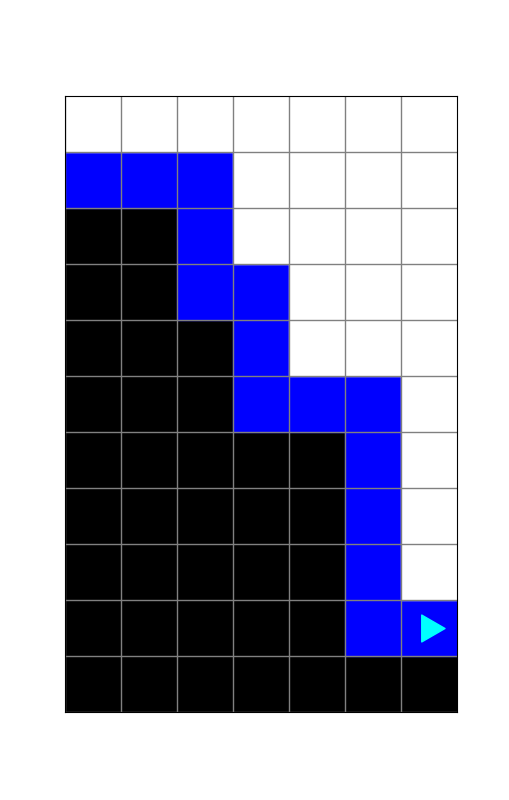
paint_the_box.py
Paint the walls of the room green.
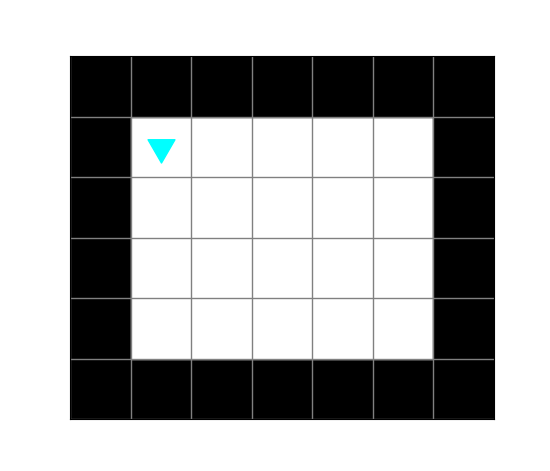
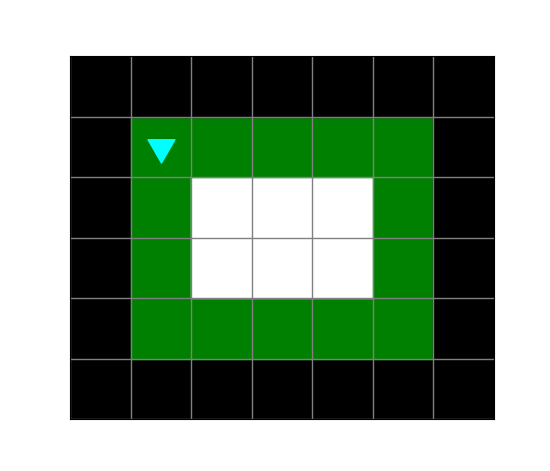
Grading
Activity | Points |
---|---|
invert.py | 5 |
invert_carefully.py | 5 |
wander.py | 5 |
fix-reds.py | 5 |
waterfall.py | 5 |
paint_the_box.py | 5 |